ストップウォッチの簡易版になります。Timerクラスを使用して、シンプルなカウントアップタイマーを作ってみたのでメモします。
完成品
解説
ポイントは次のような所かと思います。
- TimerクラスをTimer.periodic()でインスタンス化する際に、カウントアップの秒数間隔を指定
- 1秒以上の整数間隔のみカウント可能(少数指定不可=1秒未満の計測は不可)
- Timerクラスに一時停止機能なし(クラス変数でカウント情報を保持して継続することで対応)
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
bool _state = false; // 開始・停止
late Timer _countUpTimer; // << カウント情報を保持
Timer countUpTimer() {
return Timer.periodic(
const Duration(seconds: 1), // << 秒数間隔を指定
(Timer timer) {
if(_state) {
setState(() {
_counter++;
});
} else {
timer.cancel();
}
},
);
}
@override
void initState() {
super.initState();
_countUpTimer = countUpTimer();
}
...
あとはボタン押下時に_stateを切り替え、true/falseそれぞれのときに「_countUpTimer = countUpTimer();」とすればカウント開始/停止します。
以下、全文です。
import 'dart:async';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'カウントアップタイマー',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(title: 'カウントアップタイマー'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key, required this.title}) : super(key: key);
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
bool _state = false; // 開始・停止
late Timer _countUpTimer; // << カウント情報を保持
Timer countUpTimer() {
return Timer.periodic(
const Duration(seconds: 1), // << 秒数間隔を指定
(Timer timer) {
if(_state) {
setState(() {
_counter++;
});
} else {
timer.cancel();
}
},
);
}
@override
void initState() {
super.initState();
_countUpTimer = countUpTimer();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
OutlinedButton(
onPressed: () {
if (!_state) {
_countUpTimer = countUpTimer();
} else {
_countUpTimer.cancel();
}
setState(() {
_state = !_state;
});
},
child: _state ? const Icon(Icons.stop) : const Icon(Icons.play_arrow),
),
Text('${_counter.toString()}s'),
],
),
),
);
}
}
なるべく簡単に作ったので、こんな感じになりました。
参考
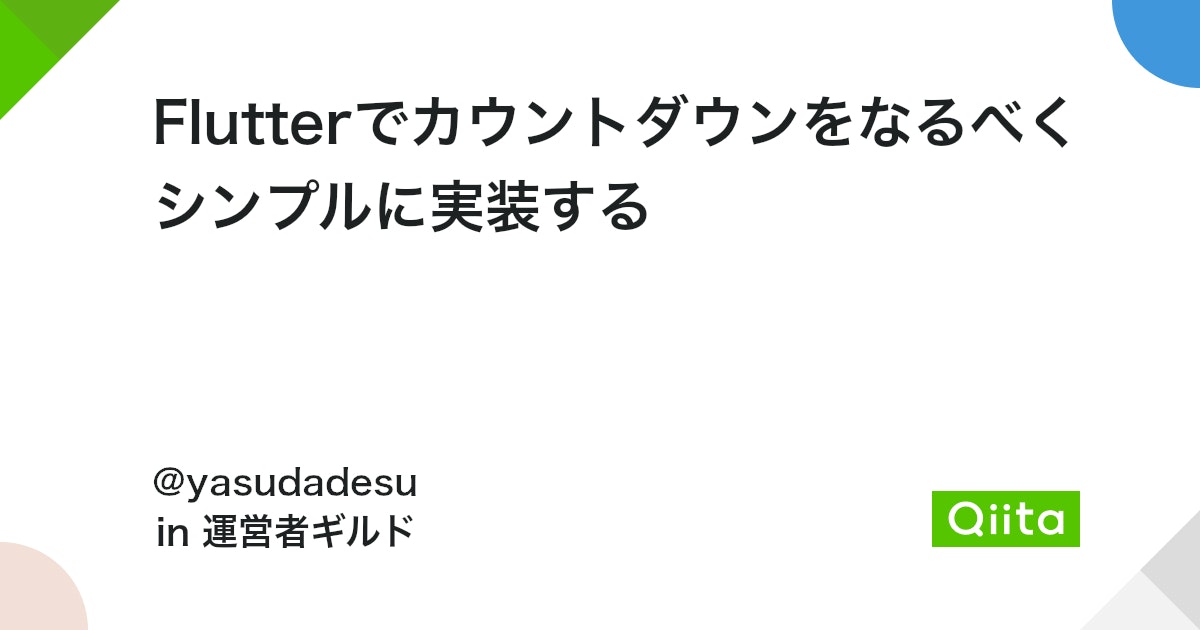
Flutterでカウントダウンをなるべくシンプルに実装する - Qiita
はじめにひょんなことからFlutterでカウントダウンするコードを書くことになったのですが、色々なサンプル実装などを見ていて以下のような引っ掛かりがありました。Timer自体にはpauseするメ…